# Implement Purchasing Power Parity for Your Overseas Product Quickly with Cloudflare Worker
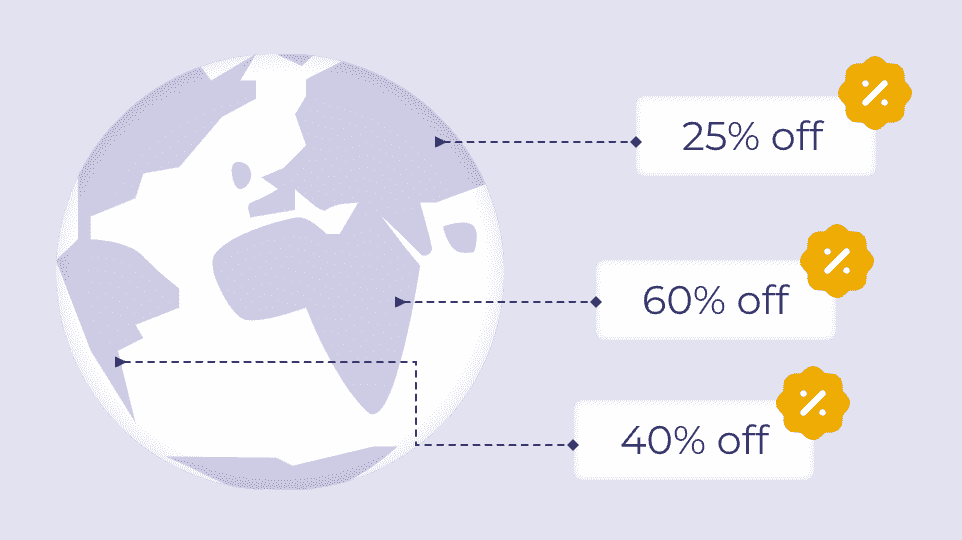
Due to different purchasing power in different countries and regions, overseas products need to set different prices for areas with varying purchasing power.
To achieve Purchasing Power Parity, you need two sets of data:
- User location data.
- A list of purchasing power levels for various countries.
The logic is simple: Location data -> Purchasing power levels -> Match and apply corresponding price information
So how do we get these two sets of data?
# Location Data
Cloudflare Worker's request parameter carries very detailed location data, allowing us to easily obtain location without the need for third-party services:
It includes latitude, longitude, region codes, etc., with the granularity down to the country level, using the country code field here.
# Purchasing Power Level Data
You can download the list of purchasing power levels for each country in this gist (opens new window).
# Logic and Implementation Code
The general logic is as follows:
- Retrieve the country code from the Worker's request parameter.
- Retrieve the purchasing power level from the list based on the country code.
- Match the level to the corresponding discount information and apply.
For simplicity in CleanClip (opens new window) (Clipboard tool on Mac), I directly apply different discounts for different countries. LemonSqueezy can apply discount codes like this: PRODUCT_URL + "?checkout%5Bdiscount_code%5D=" + discountCode
Some details:
- Discount information is saved in environment variables for easy updating.
- Access-Control-Max-Age cache is set to 0 for instant changes and effects (not setting it may result in lasting previous results, typically taking about 3-4 days to update, setting it to 0 updates immediately).
- You can connect this worker to another worker below it, maintaining price information centrally here for ease of use across multiple pages and businesses.
import ppp from "./pppdata.js";
// Map the list of purchasing power data for easy searching
const flatppp = ppp.flatMap(category => category.countries.map( countryInfo => {
return {
range: category.range,
countryCode: countryInfo.country,
countryName: countryInfo.countryName
}
}))
// Find the corresponding country in the purchasing power level list
function findCountry(countryCode) {
return flatppp.find(deal => deal.countryCode == countryCode)
}
// Get the discount information configured in the environment variables based on the purchasing power level
function getDiscount(env, range) {
switch(range) {
// Cases for different ranges with corresponding discount information
}
}
// Merge country's purchasing power information with discount information
function mergeDiscountResult(countryPPP, discount) {
return JSON.stringify({
range: countryPPP.range,
countryCode: countryPPP.countryCode,
countryName: countryPPP.countryName,
discountCode: discount.code,
discount: discount.discount
});
}
// Construct the response
function responseFor(result, code) {
return new Response(result, {
status: code,
headers: {
"Content-Type": "application/json",
"Access-Control-Allow-Origin": "*",
"Access-Control-Allow-Headers": "*",
"Access-Control-Allow-Methods": "GET, OPTIONS, POST, PUT, DELETE",
"Access-Control-Max-Age": "0"
}
});
}
// ✨ Core code
export default {
async fetch(request, env, ctx) {
// 1. Get the country code
const countryCode = request.cf.country
// 2. Find the country in the purchasing power list
let countryPPP = findCountry(countryCode)
// 3. Get the corresponding discount information based on the country's purchasing power
let discount = getDiscount(env, countryPPP.range)
if (countryPPP && discount) {
// Construct the result
let result = mergeDiscountResult(countryPPP, discount)
// 4. Can directly return the result for other services to call
return responseFor(result, 200)
} else {
return responseFor("Error", 500)
}
// 5. Or directly 301 redirect to the specified discount link
// let url = env.TARGET_DOMAIN
// if (discountCode !== undefined && discountCode.length > 0) {
// url = env.TARGET_DOMAIN + "?checkout%5Bdiscount_code%5D=" + discountCode
// }
// var response = Response.redirect(url, 301);
},
};
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
Author: Sintone Li
Article link: https://cleanclip.cc/gb/developer/cloudflare-worker-implements-purchasing-power-parity/